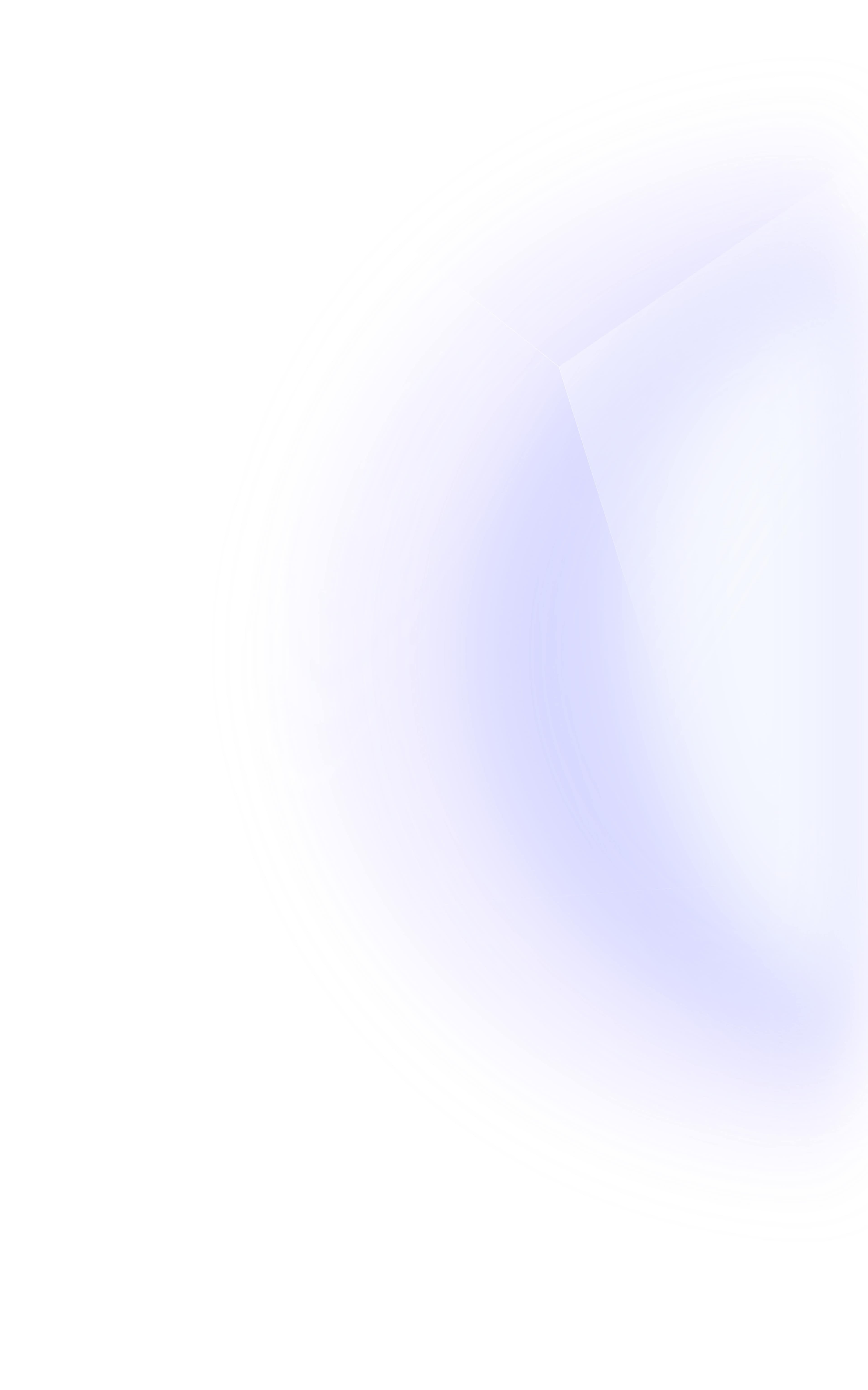
The Complete Guide to Solana Smart Contracts

Written by Adesoye Jeremiah Olayinka

Reviewed by Logan Ross
Solana is a blockchain platform that focuses on smart contract development and aims to address the challenges faced by existing blockchains. It hosts various blockchain projects, including NFTs and DeFi projects.
The Solana ecosystem has grown in popularity in recent years thanks to its incredibly low transaction fees and high scalability. What sets Solana apart is its combination of the proof of stake consensus algorithm with a unique proof of history mechanism, providing developers with a versatile network for creating NFT platforms, dApps, and more.
This article explains how Solana smart contracts work, how to create and deploy Solana programs, and the necessary tools. Furthermore, we will review some Solana smart contract tutorials, examples, repositories, developer tools, and resources to get you started.
What is a Solana smart contract?
Solana smart contracts, also known as programs, are codes that interpret instructions and establish the terms of an agreement. Many developers consider Solana smart contracts a viable alternative to Ethereum smart contracts, thanks to the comparatively low transaction fees.
Smart contracts enable developers to create apps that leverage blockchain security, dependability, and accessibility while providing sophisticated peer-to-peer functionality ranging from loans and insurance to logistics and gaming.
Solana smart contracts can be written in a variety of programming languages. While Rust is the native Solana smart contract language, the protocol also supports smart contract development in C++, Solidity, and other languages via third-party JSON RPC API SDK clients.
Solana Smart Contract Architecture
Solana uses a different smart contract model than traditional EVM-based blockchain. In the case of traditional blockchains, the code and state are combined into a single contract, whereas Solana smart contracts are stateless and contain only program logic.
The Solana architecture seeks to demonstrate the existence of a set of software algorithms that, when combined to implement a blockchain, eliminate software as a performance bottleneck, allowing transaction throughput to scale proportionally with network bandwidth.
To enhance interaction with dApps, Solana also comes with a Command Line Interface (CLI) and JSON RPC API. dApps can also interact with the Blockchain and Solana programs using existing SDKs.
Diagram of the Solana validation process
Solana's Proof-of-History Mechanism
Solana aims to improve blockchain scalability by combining proof of stake with proof of history. It uses a Tower Byzantine fault-tolerant (BFT) system, which eliminates the need for nodes to communicate with one another in real-time, resulting in increased efficiency and a high transaction rate of 50,000 transactions per second (TPS).
As a result, Solana can handle high transaction volume while maintaining decentralization. Solana creates new blocks every 400 milliseconds with the assistance of 200 validating nodes, all while keeping transaction fees under a dollar.
Solana is known for its increased scalability and faster transaction speed. The Solana network is scalable at the core level, so it does not require layer-2 solutions to increase scalability.
The Solana network's technology breaks down data into smaller chunks, making it easier to transfer data across the network. Solana also uses Sealevel to aid in processing transactions across GPUs and SSDs, resulting in an efficient blockchain network.
Image credit: Solana Labs
What are the differences between a Solana Program and an Ethereum smart contract?
Ethereum's smart contracts are written in the Solidity programming language, while Solana's smart contracts are primarily written in Rust and are referred to as programs. They are stateless and only represent program logic.
The most significant difference between the two is in terms of the consensus mechanism that is employed. Ethereum uses Proof of Stake (PoS), aiming for a more decentralized and energy-efficient network. On the other hand, Solana utilizes Proof of History (PoH), a unique approach that prioritizes speed and efficiency, resulting in fast and low-cost transactions.
A Solana program is made up entirely of code and contains no data. All data is fed in as inputs. This decoupled design enables high performance by allowing many copies of a Solana program to run in parallel on different inputs. In other words, transactions from multiple user accounts to the same Solana program can occur concurrently. This is one of the features that make Solana highly scalable.
How to Create a Smart Contract on Solana
This section delves into the steps necessary to create a smart contract on Solana. We’ll guide you through the process and provide all the information you need to get started.
Prerequisite
Little knowledge of how Solana works
Anchor and Rust installed in accordance with the Setup section below
Readiness to learn
Step 1: Install Rust, Solana, Yarn and Anchor
Let’s get started with setting up the Solana development environment.
Install Rust
We will start by installing Rust, the programming language used for Solana programs.
Verify if Rust and the Rust compiler were installed correctly using the following commands.
You should also now have Cargo, the Rust package manager, installed. Run the command below to confirm that Cargo was installed correctly.
Rustup is the tool that installs and updates Rust.
Rustc is the compiler of the Rust programming language; It enables you to take a Rust program and make it executable on all current operating systems.
Cargo is the tool that builds systems and manages packages for the Rust programming language.
Install Solana
Follow the instructions if the installation asks you to change your path.
Install Node.js
Visit the official website to download Node.js, ensuring you select the appropriate version for your operating system.
You can confirm the installation of Node.js by running the following commands:
Install Yarn
Yarn is a package manager like npm.
Check your Yarn version.
Install Anchor
Anchor is a framework for Solana's Sealevel runtime that offers several useful developer tools.
To install Anchor run the following command.
Then, confirm the installation.
Step 2: Set up the Project
In this section we configure our solana cli to devnet as well as initialize a project with the anchor framework. To simulate actual deployment, we will deploy to the Solana Devnet.
To proceed, run the following command in your terminal.
The next step is to create a wallet, which is necessary for running and distributing your programs.
Retrieve your pubkey with the following command.
After that, you can query your address by running the following command.
Then, you will need some testnet SOL for development. You can airdrop some to your wallet using the following command.
Running the command below will display your balance.
Let’s initialize our Anchor project by running the command below. The command will create all folders and extra dependencies needed for the project:
Step 3: Write our First HelloWorld Program
In the project structure, you will see the following files and folders.
program — This is the directory containing all of your Solana programs
test — A folder for Javascript test code
migrations — This is the deploy script for the program
app — The location for building our frontend
Remember that lib.rs is the starter and main file for our Solana program. It has some starter codes, as shown below.
Let’s explore how Rust works.
The first line of code in Rust imports dependencies or libraries. It is importing the anchor library in this case.
Solana stores the address or program ID in the declare_Id variable. Anchor creates a program ID for us by default.
Next is the program section, where the logic of the program lives.
The last section is the derive Accounts section, where the account struct lives.
The initialize struct defines the context of the initialize function, and a struct is used to declare a structure. Defining methods in Rust is different from other programming languages. Solana's ability to separate code and data is one of its key selling points.
Let's dive into the Hello World Solana program. Keep in mind that Solana programs are just special accounts on the Solana network that can store and carry out instructions. We'll be using the solana-program crate, which is like a standard library for Solana programs. We'll need to use some things from the solana-program crate to make a basic program.
The first section uses Borsh, which stands for Binary Object Representation Serializer for hashing. Borsh is used in serializing and deserializing parameters passed to and from the deployed program. AccountInfo is a struct in the account _info module that provides access to account information. The entrypoint declares the program's entry point.
The ProgramResult within the entrypoint module returns the ProgramReport or ProgramError. Finally, the msg, another macro, functions in printing messages to the program log, whereas the struct pubkey allows us to access addresses as a public key.
The code snippet above defines the type of state stored in the account and the counter u32, which is the 32-bit unsigned integer type referencing the number of greetings.
Solana programs require an entrypoint macro to process program instructions, as seen in the first line of code above. The entrypoint will require a process_instruction function with program_id argument, which is the account's public key producing the hello world program. The accounts argument is the account we intend to say hello to, and the instruction_data argument contains additional inputs. The ProgramResult prints a message "Hello World Rust Program."
The 'let' statement gets the accounts to greet. The 'if' statement states the condition that for data to be modified, the program must own the account.
The 'let' statement gets the accounts to greet. The 'if' statement states the condition that for data to be modified, the program must own the account.
How to Deploy a Smart Contract on Solana
This section outlines the steps to deploy a smart contract on Solana.
Step 1: Testing the Solana program
To run the tests, execute the following command.
This will build, deploy, and test programs against a specific cluster.
Step 2: Deploying to Devnet
Deploying to a live network is easy, we have to first confirm we are on Devnet.
Locate your Anchor.toml and update the cluster to devnet cluster = "devnet".
Build the program by running the following command.
Lastly, deploy the program.
Step 3: Verify on the Solana Explorer
Next, we authenticate the program on the Solana Devnet explorer to verify it was successfully deployed by providing the program id. You should see the deployment log under the history section.
Solana Smart Contract Tutorials
Now that we have a solid understanding of how smart contracts work, let's dive into a real-world project to master how the Solana development architecture works. The project we will be building is a Solana smart contract for minting NFTs. We can mint NFTs in various ways, one of which is using the Metaplex candy machine. NFTs are one of the most popular use cases of smart contracts.
In this section, you'll learn
How to configure the Solana CLI utility to use Devnet and other useful commands in Solana
How to write more complex programs in Rust
How to init a project with anchor framework
Understanding Rust variables and syntax
The requirements are
The latest stable Rust build
Solana CLI v1.7.11 or later
Quick setup
We configure the development environment using the command below to set up Solana to work with the Devnet network.
Then generate your wallet, which will be required to run and deploy your programs using the command below.
You'll be prompted to enter a password to secure your wallet. Then you'll see your mnemonic, which is a combination of 12 words:
You can then check your address.
Then, run the command below to Airdrop 4 testnet SOL to your address.
After aidropping the 4 testnet SOL, check your balance by running the following command.
Now that you've configured Solana to work with the Devnet network and created a new wallet, let's create an Anchor project to manage all the folders and tedious configurations for us. Go to your terminal and type the following command.
The Solana Smart Contract
Before diving into the smart contract, we need to set up our spl-token CLI that will be used to interact with SPL tokens. You can do that by running the command below in your terminal.
Install solana sdk and solana-program.
You'll notice a lib.rs file in the programs folder. This is where our Solana program will be written. As mentioned earlier, we would be building on the default given in the lib.rs. You should see something like this.
Here, we imported the tools we would be working with at the top of our code.
Defining Our Program’s Logic
We are giving authority to the program using mint_authority to create a mint (mint_nft) and then assigning a token account to it (mint_token_account). Then we will work with the token_program and associated_token_program that we imported earlier.
Authority means that the account can mint more tokens or print more money. It is usually created by the wallet account. It is optional because a mint authority can revoke its own right, rendering the mint account immutable.
Creating mint account
Creating token account
Writing the Mint Function
Next, we will mint the NFT to the token account we created above.
Deploying the Program
You’re now ready to build the NFT minting Solana program using the Anchor framework!
The build process generates the key pair for your program’s account. Before you deploy your program, you must add this public key to your lib.rs file, as it’s required by programs that use Anchor. To do this, you need to get the key pair from the keypair.json file that was generated by Anchor using the following command.
The next step is to edit the lib.rs file and replace the key pair in the declare_id!() definition with the value you obtained from the previous step.
Next, you also need to insert the obtained Program ID value into the Anchor.toml file in the chainlink_solana_demo devnet definition.
You might want to build the program again using Anchor because you have replaced the keypair with a new ID.
Finally, you can deploy the program.
Once the program has been successfully deployed, the terminal output will show the program ID, which should correlate with the value you entered into the lib.rs and Anchor.toml files.
The deployed contact can also be verified on the Solana Devnet Explorer.
The deployed contract is shown on the Solana Devnet explorer.
Solana Smart Contract Examples and Repos
There are numerous Solana smart contract examples and repositories that would be of great value in your Solana development journey. We have outlined some of them below.
1. Hello World Smart Contract
Hello World smart contract provides a hands-on Solana program example using Anchor and Rust. It follows a step-by-step approach to building smart contracts and dApps in Solana. It also provides sample codes for using, building, and deploying on-chain programs.
2. Simple Solana Program
Simple Solana Program is functionally related to the Hello World example. It demonstrates how to create and invoke a program on the Solana blockchain. In this example, the Solana program counts the number of times it has been executed and stores that information on-chain.
3. Awesome Solana
Awesome Solana is a detailed library containing resources for beginners and advanced Solana developers. It provides resources on Solana development, Solana transactions, Solana libraries, explanations of how Solana programs work, and video examples to aid your learning.
4. Break Solana
Break Solana is a game that consists of a web client frontend, a web server backend, and an on-chain Solana program. The Break Solana game allows a player to send simple, smart contract transactions as quickly as possible to demonstrate Solana's speed.
Solana Developer Tools and Resources for Writing Programs
There is a collection of learning resources and developer tools which is useful while writing programs on Solana.
1. Solana Playground
Solana Playground offers a super easy way for users to interact with the blockchain system by allowing them to fully customize Solana transactions according to their unique needs. Even those with no experience developing computer programs can use Solana Playground to better understand how the blockchain operates. The project’s repository is available on Github.
2. Start on Solana
Start on Solana offers guidance for building on Solana and contributing to the Solana ecosystem. It offers various quests in Javascript and Rust, from creating an NFT on Solana to minting and creating cryptocurrencies with Javascript.
3. Solana Cookbook
Solana Cookbook is a developer resource that provides the fundamental concepts and references for building applications on Solana. It is an essential resource that focuses on Solana development while providing lots of examples for developers.
4. Sol Dev Course
The Sol Dev Course is designed to simplify the Solana development experience, making it one of the best places for beginners to start building on Solana. It also has an engaging community, allowing members to contribute content to help other web3 developers.
5. Build a Web3 app on Solana with React and Rust by Buildspace
Buildspace is an amazing community to begin your Solana development journey as well as earn NFTs after completing a milestone. The interactive courses are perfect for beginners who want to build things quickly on Solana.
Start Writing Solana Programs
Navigating the world of blockchain and smart contracts can be intricate, but platforms like Solana are paving the way for more efficient, scalable, and cost-effective solutions. This guide has provided a comprehensive overview of Solana's unique approach to smart contract development, emphasizing its distinct architecture, consensus mechanisms, and the tools available for developers.
Whether you're a seasoned developer or just starting in the blockchain space, Solana offers a promising avenue for innovation. As the blockchain landscape evolves, platforms like Solana will undoubtedly play a pivotal role in shaping the future of decentralized applications and the broader web3 ecosystem. Dive in, experiment, and harness the power of Solana for your next project.
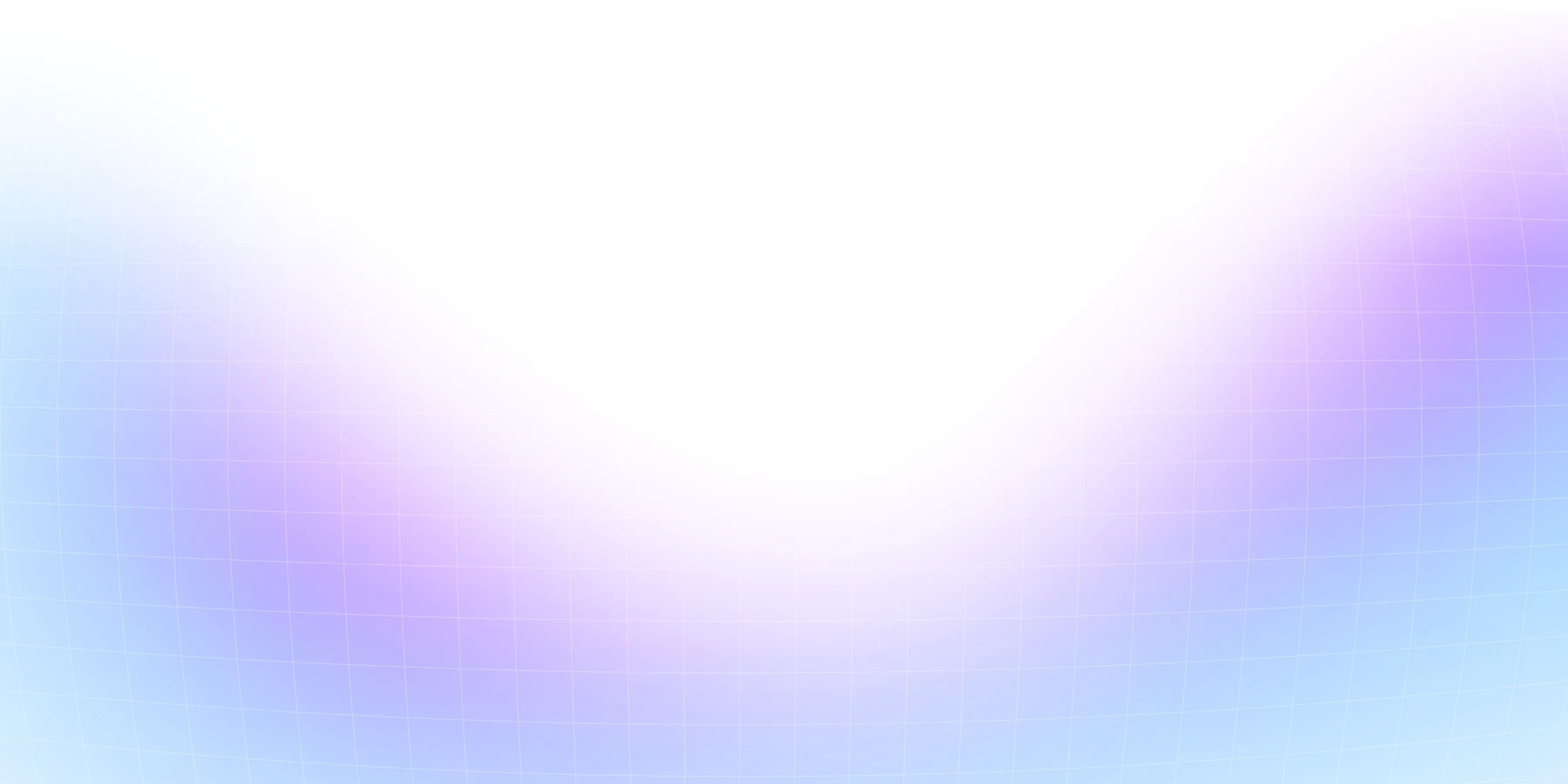
Related overviews
Learn About Compressed NFTs and How They Work
Learn What an Associated Token Account Is, How it Works, and How to Create One
Learn What SFTs Are, How They Work, and What Makes Them Different from NFTs and SPL Tokens