How to Mint an NFT with Ethers.js
This tutorial describes how to mint an NFT on the Ethereum blockchain using Ethers via the ethers.js library, and our smart contract from Part I: How to Create an NFT. We'll also explore basic test se
Estimated time to complete this guide: ~10 minutes
Plus, be sure to check out the rest of our NFT tutorial series:
In another tutorial, we learned how to mint an NFT using Web3 and the OpenZeppelin contracts library. In this exercise, we're going to walk you through an alternative implementation using version 4 of the OpenZeppelin library as well as the Ethers.js Ethereum library instead of Web3.
We'll also cover the basics of testing your contract with Hardhat and Waffle. For this tutorial I'm using Yarn, but you can use npm/npx if you prefer.
Lastly, we'll use TypeScript. This is fairly well documented, so we won't cover it here.
In all other respects, this tutorial works the same as the Web3 version, including tools such as Pinata and IPFS.
A Quick Reminder
As a reminder, "minting an NFT" is the act of publishing a unique instance of your ERC721 token on the blockchain. This tutorial assumes that that you've successfully deployed a smart contract to the Ropsten network in Part I of the NFT tutorial series, which includes installing Ethers.
Step 1: Create your Solidity contract
OpenZeppelin is library for secure smart contract development. You simply inherit their implementations of popular standards such as ERC20 or ERC721, and extend the behavior to your needs. We're going to put this file at contracts/MyNFT.sol.
// Contract based on https://docs.openzeppelin.com/contracts/4.x/erc721
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC721/extensions/ERC721URIStorage.sol";
import "@openzeppelin/contracts/utils/Counters.sol";
contract MyNFT is ERC721URIStorage {
using Counters for Counters.Counter;
Counters.Counter private _tokenIds;
constructor() ERC721("MyNFT", "MNFT") {}
function mintNFT(address recipient, string memory tokenURI)
public
returns (uint256)
{
_tokenIds.increment();
uint256 newItemId = _tokenIds.current();
_mint(recipient, newItemId);
_setTokenURI(newItemId, tokenURI);
return newItemId;
}
}
Step 2: Create Hardhat tasks to deploy our contract and mint NFT's
Create the file tasks/nft.ts containing the following:
import { task, types } from "hardhat/config";
import { Contract } from "ethers";
import { TransactionResponse } from "@ethersproject/abstract-provider";
import { env } from "../lib/env";
import { getContract } from "../lib/contract";
import { getWallet } from "../lib/wallet";
task("deploy-contract", "Deploy NFT contract").setAction(async (_, hre) => {
return hre.ethers
.getContractFactory("MyNFT", getWallet())
.then((contractFactory) => contractFactory.deploy())
.then((result) => {
process.stdout.write(`Contract address: ${result.address}`);
});
});
task("mint-nft", "Mint an NFT")
.addParam("tokenUri", "Your ERC721 Token URI", undefined, types.string)
.setAction(async (tokenUri, hre) => {
return getContract("MyNFT", hre)
.then((contract: Contract) => {
return contract.mintNFT(env("ETH_PUBLIC_KEY"), tokenUri, {
gasLimit: 500_000,
});
})
.then((tr: TransactionResponse) => {
process.stdout.write(`TX hash: ${tr.hash}`);
});
});
Step 3: Create helpers
You'll notice our tasks imported a few helpers. Here they are.
contract.ts
env.ts
export function env(key: string): string {
const value = process.env[key];
if (value === undefined) {
throw `${key} is undefined`;
}
return value;
}
provider.ts
Note that the final getProvider() function uses the ropsten network. This argument is optional and defaults to "homestead" if omitted. We're using Alchemy of course, but there are several supported alternatives.
import { ethers } from "ethers";
export function getProvider(): ethers.providers.Provider {
return ethers.getDefaultProvider("ropsten", {
alchemy: process.env.ALCHEMY_API_KEY,
});
}
wallet.ts
import { ethers } from "ethers";
import { env } from "./env";
import { getProvider } from "./provider";
export function getWallet(): ethers.Wallet {
return new ethers.Wallet(env("ETH_PRIVATE_KEY"), getProvider());
}
Step 4: Create tests
Under your test directory, create these files. Note that these tests are not comprehensive. They test a small subset of the ERC721 functionality offered by the OpenZeppelin library, and are intended to provide you with the building blocks to create more robust tests.
test/MyNFT.spec.ts (unit tests)
tasks.spec.ts (integration specs)
import { deployTestContract, getTestWallet } from "./test-helper";
import { waffle, run } from "hardhat";
import { expect } from "chai";
import sinon from "sinon";
import * as provider from "../lib/provider";
describe("tasks", () => {
beforeEach(async () => {
sinon.stub(provider, "getProvider").returns(waffle.provider);
const wallet = getTestWallet();
sinon.stub(process, "env").value({
ETH_PUBLIC_KEY: wallet.address,
ETH_PRIVATE_KEY: wallet.privateKey,
});
});
describe("deploy-contract", () => {
it("calls through and returns the transaction object", async () => {
sinon.stub(process.stdout, "write");
await run("deploy-contract");
await expect(process.stdout.write).to.have.been.calledWith(
"Contract address: 0x610178dA211FEF7D417bC0e6FeD39F05609AD788"
);
});
});
describe("mint-nft", () => {
beforeEach(async () => {
const deployedContract = await deployTestContract("MyNFT");
process.env.NFT_CONTRACT_ADDRESS = deployedContract.address;
});
it("calls through and returns the transaction object", async () => {
sinon.stub(process.stdout, "write");
await run("mint-nft", { tokenUri: "https://example.com/record/4" });
await expect(process.stdout.write).to.have.been.calledWith(
"TX hash: 0xd1e60d34f92b18796080a7fcbcd8c2b2c009687daec12f8bb325ded6a81f5eed"
);
});
});
});
test-helpers.ts
Note this require the NPM libraries imported, including sinon, chai, and sinon-chai. The sinon.restore()
call is necessary due to the use of stubbing.
Step 5: Configuration
Here's our fairly bare bones hardhat.config.ts
.
import("@nomiclabs/hardhat-ethers");
import("@nomiclabs/hardhat-waffle");
import dotenv from "dotenv";
// You need to export an object to set up your config
// Go to https://hardhat.org/config/ to learn more
const argv = JSON.parse(env("npm_config_argv"));
if (argv.original !== ["hardhat", "test"]) {
require('dotenv').config();
}
import("./tasks/nft");
import { HardhatUserConfig } from "hardhat/config";
const config: HardhatUserConfig = {
solidity: "0.8.6",
};
export default config;
Note the conditional to only invoke dotenv if we're not running tests. You might not want to run this in production, but rest assured that dotenv will silently ignore it if the .env file isn't present.
Running Our Tasks
Now that we've put these files in place, we can run hardhat to see our tasks (excluding the built-in tasks for brevity).
AVAILABLE TASKS:
deploy-contract Deploy NFT contract
mint-nft Mint an NFT
Forget the arguments to your task? No problem.
$ hardhat help deploy-contract
Usage: hardhat [GLOBAL OPTIONS] deploy-contract
deploy-contract: Deploy NFT contract
Running Our Tests
To run our tests, we run hardhat test
.
mintNft
✓ calls through and returns the transaction object (60ms)
MyNFT
mintNft
✓ emits the Transfer event (60ms)
✓ returns the new item ID
✓ increments the item ID (57ms)
✓ cannot mint to address zero
balanceOf
✓ gets the count of NFTs for this address
6 passing (2s)
✨ Done in 5.66s.
Summary
In this tutorial, we've created a firm foundation for a well tested NFT infrastructure based on Solidity. The wallet provided by waffle.provider.getWallets() links to a local fake Hardhat Network account that conveniently comes preloaded with an eth balance that we can use to fund our test transactions.
Related articles
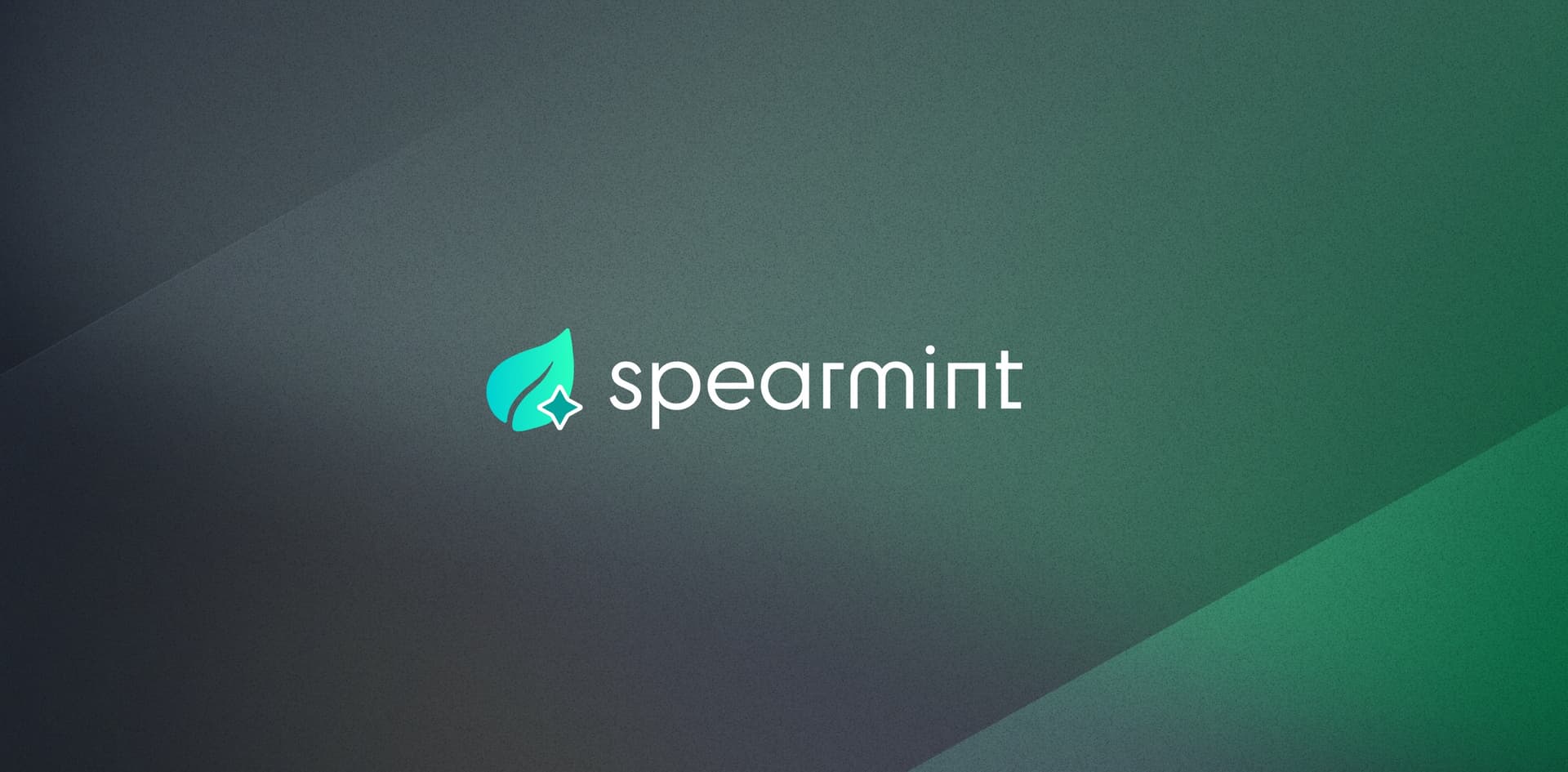
Spearmint, the Free and Automated Allowlist Platform
Elevate your NFT game with Spearmint, the free automated allowlist platform. Manage NFT minting, foster community engagement, and avoid costly gas wars.
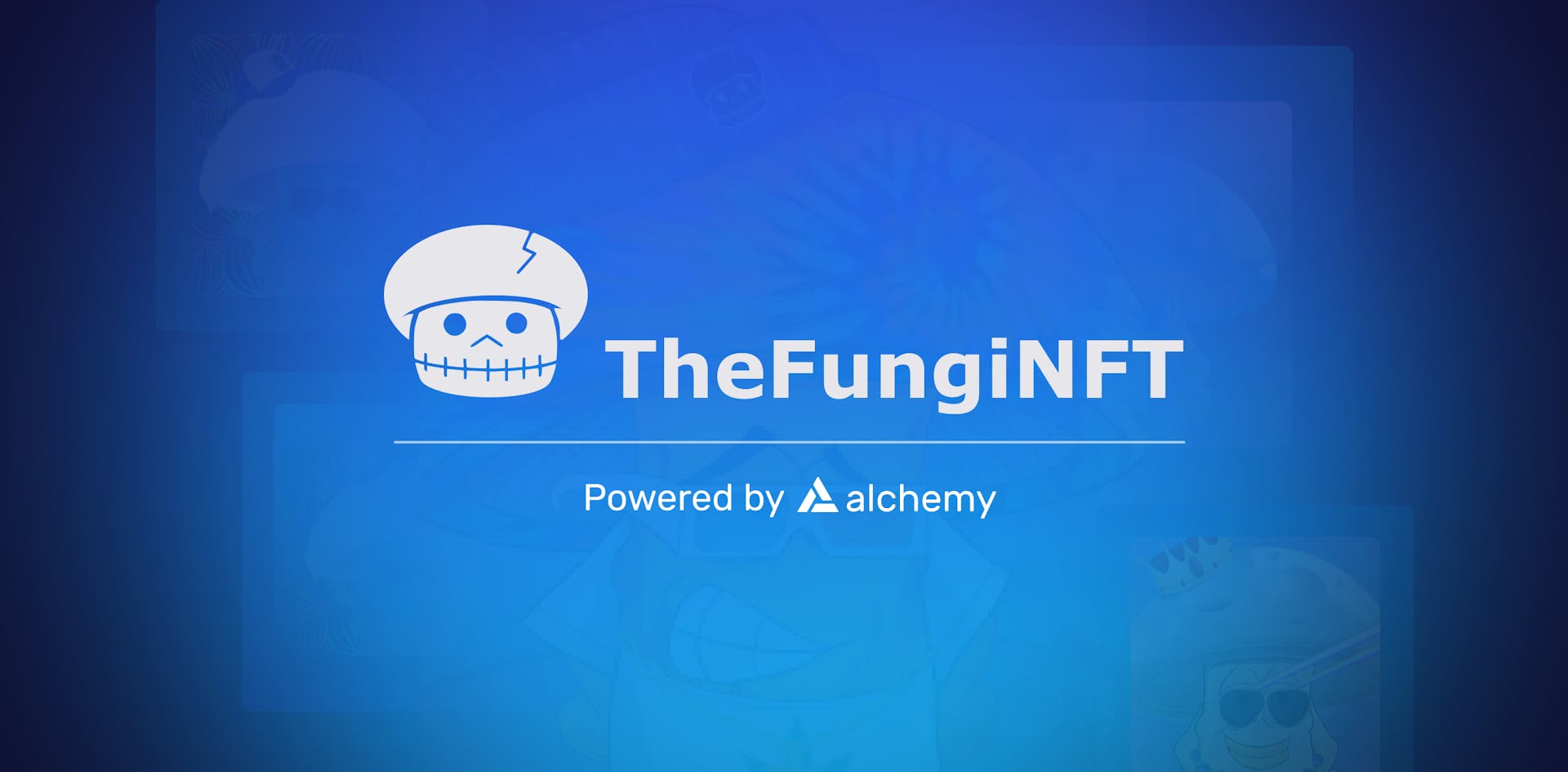
TheFungiNFT Partners with Alchemy to Leverage NFT Drops for Social Good
TheFungiNFT collaborates with Alchemy for impactful NFT drops, and help raise awareness for mental health.
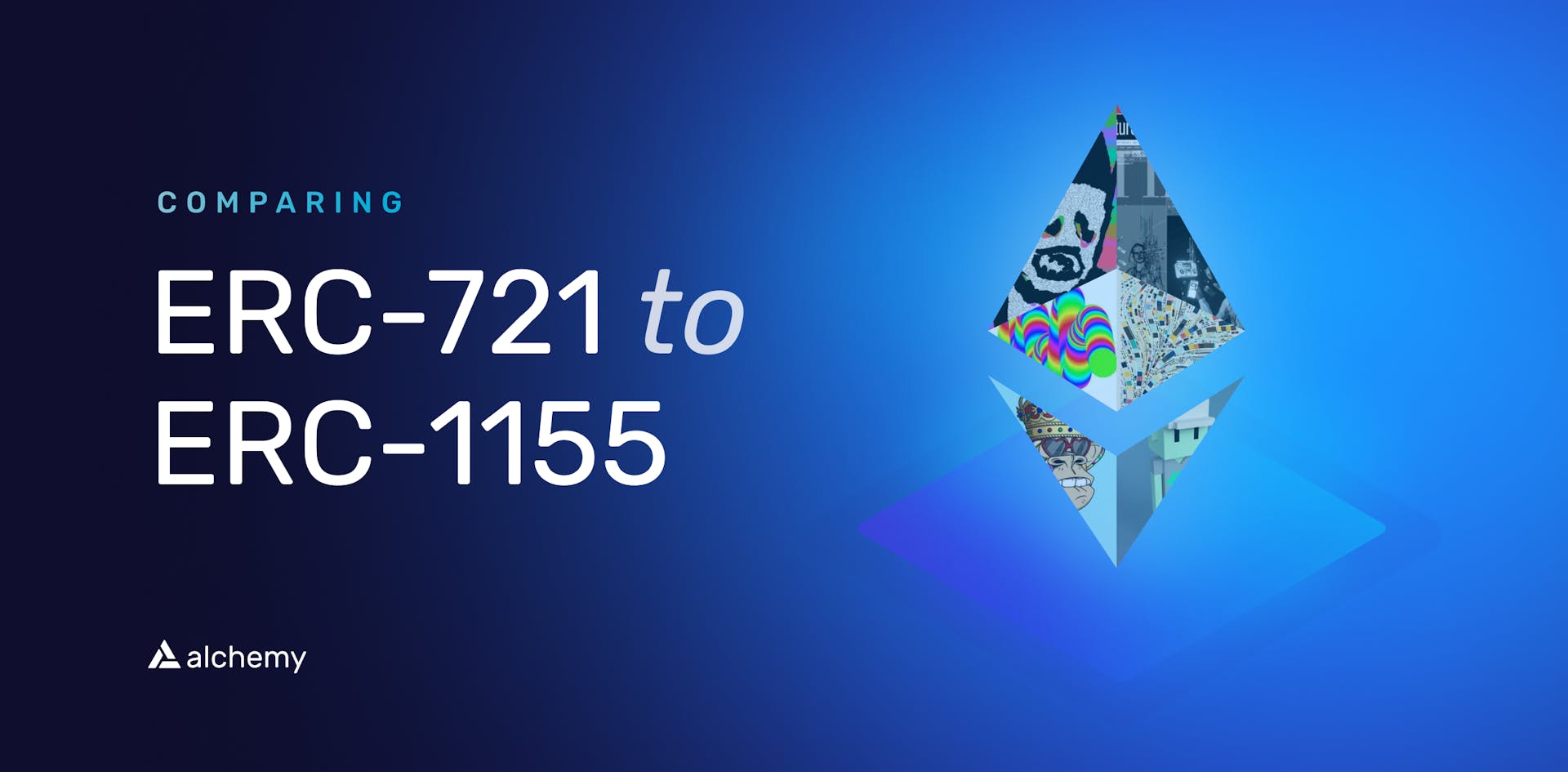
Your Guide to ERC-1155: Comparing ERC-721 to ERC-1155
Learn about the ERC-1155 token standard and compare ERC-721 vs. ERC-1155, so you can understand the differences and when to employ each.
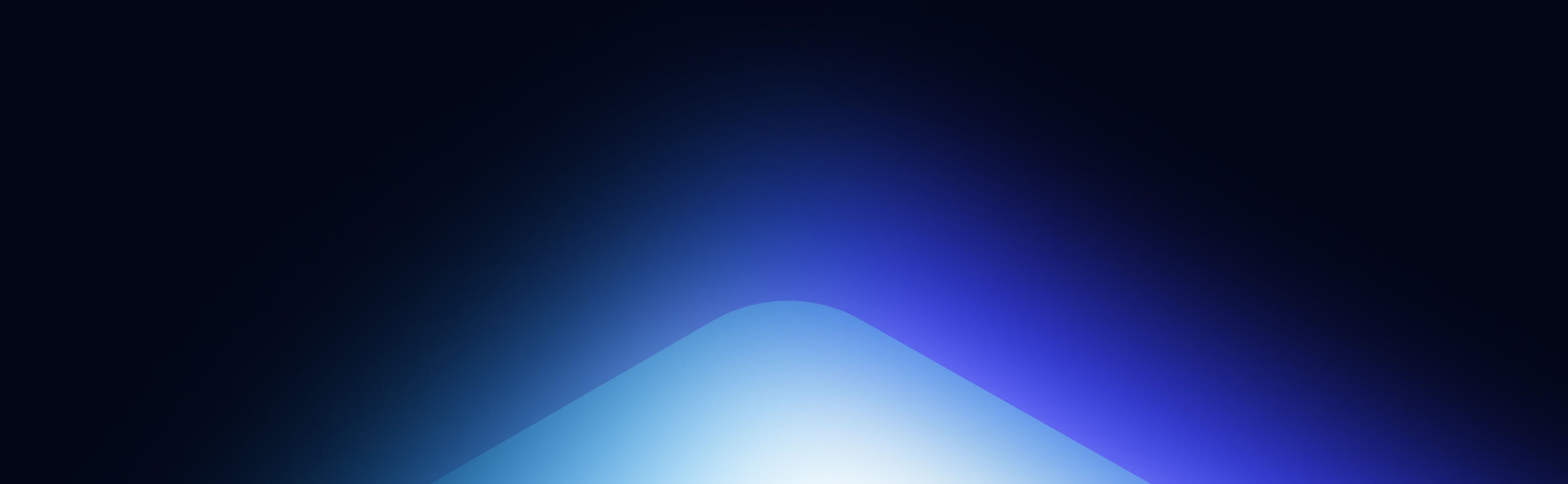